|
virtual const ProcessorInfo | getProcessorInfo () const override |
|
| VolumeGLProcessor (std::shared_ptr< const ShaderResource > fragmentShader, bool buildShader=true) |
|
| VolumeGLProcessor (const std::string &fragmentShader, bool buildShader=true) |
|
virtual void | process () override |
|
| Processor (const std::string &identifier="", const std::string &displayName="") |
|
std::string | getClassIdentifier () const |
|
std::string | getCategory () const |
|
CodeState | getCodeState () const |
|
Tags | getTags () const |
|
void | setIdentifier (const std::string &identifier) |
|
const std::string & | getIdentifier () const |
|
void | setDisplayName (const std::string &displayName) |
|
const std::string & | getDisplayName () const |
|
virtual std::vector< std::string > | getPath () const override |
|
virtual void | setProcessorWidget (std::unique_ptr< ProcessorWidget > processorWidget) |
|
ProcessorWidget * | getProcessorWidget () const |
|
bool | hasProcessorWidget () const |
|
virtual void | setNetwork (ProcessorNetwork *network) |
|
ProcessorNetwork * | getNetwork () const |
|
virtual void | initializeResources () |
|
Port * | getPort (const std::string &identifier) const |
|
Inport * | getInport (const std::string &identifier) const |
|
Outport * | getOutport (const std::string &identifier) const |
|
const std::vector< Inport * > & | getInports () const |
|
const std::vector< Outport * > & | getOutports () const |
|
const std::string & | getPortGroup (Port *port) const |
|
std::vector< std::string > | getPortGroups () const |
|
const std::vector< Port * > & | getPortsInGroup (const std::string &portGroup) const |
|
const std::vector< Port * > & | getPortsInSameGroup (Port *port) const |
|
bool | allInportsConnected () const |
|
bool | allInportsAreReady () const |
|
bool | isSource () const |
|
bool | isSink () const |
|
bool | isReady () const |
|
virtual void | doIfNotReady () |
|
virtual void | setValid () override |
|
virtual void | invalidate (InvalidationLevel invalidationLevel, Property *modifiedProperty=nullptr) override |
|
void | addInteractionHandler (InteractionHandler *interactionHandler) |
|
void | removeInteractionHandler (InteractionHandler *interactionHandler) |
|
bool | hasInteractionHandler () const |
|
const std::vector< InteractionHandler * > & | getInteractionHandlers () const |
|
virtual void | invokeEvent (Event *event) override |
|
virtual void | propagateEvent (Event *event, Outport *source) override |
|
virtual Processor * | getProcessor () override |
|
virtual const Processor * | getProcessor () const override |
|
virtual void | serialize (Serializer &s) const override |
|
virtual void | deserialize (Deserializer &d) override |
|
template<typename T , typename std::enable_if_t< std::is_base_of< Inport, T >::value, int > = 0> |
T & | addPort (std::unique_ptr< T > port, const std::string &portGroup="default") |
|
template<typename T , typename std::enable_if_t< std::is_base_of< Outport, T >::value, int > = 0> |
T & | addPort (std::unique_ptr< T > port, const std::string &portGroup="default") |
|
template<typename T > |
T & | addPort (T &port, const std::string &portGroup="default") |
|
Port * | removePort (const std::string &identifier) |
|
Inport * | removePort (Inport *port) |
|
Outport * | removePort (Outport *port) |
|
virtual | ~PropertyOwner () |
| Removes all properties and notifies its observers of the removal.
|
|
virtual void | addProperty (Property *property, bool owner=true) |
|
virtual void | addProperty (Property &property) |
|
template<typename... Ts> |
void | addProperties (Ts &... properties) |
|
virtual void | insertProperty (size_t index, Property *property, bool owner=true) |
| insert property property at position index If index is not valid, the property is appended. More...
|
|
virtual void | insertProperty (size_t index, Property &property) |
| insert property property at position index If index is not valid, the property is appended. More...
|
|
virtual Property * | removeProperty (const std::string &identifier) |
|
virtual Property * | removeProperty (Property *property) |
|
virtual Property * | removeProperty (Property &property) |
|
virtual Property * | removeProperty (size_t index) |
| remove property referred to by index More...
|
|
const std::vector< Property * > & | getProperties () const |
|
const std::vector< CompositeProperty * > & | getCompositeProperties () const |
|
std::vector< Property * > | getPropertiesRecursive () const |
|
Property * | getPropertyByIdentifier (const std::string &identifier, bool recursiveSearch=false) const |
|
Property * | getPropertyByPath (const std::vector< std::string > &path) const |
|
template<class T > |
std::vector< T * > | getPropertiesByType (bool recursiveSearch=false) const |
|
size_t | size () const |
|
Property * | operator[] (size_t) |
|
const Property * | operator[] (size_t) const |
|
iterator | begin () |
|
iterator | end () |
|
const_iterator | cbegin () const |
|
const_iterator | cend () const |
|
virtual bool | isValid () const |
|
InvalidationLevel | getInvalidationLevel () const |
|
void | setAllPropertiesCurrentStateAsDefault () |
|
void | resetAllPoperties () |
|
virtual InviwoApplication * | getInviwoApplication () |
|
void | notifyObserversWillAddProperty (Property *property, size_t index) |
|
void | notifyObserversDidAddProperty (Property *property, size_t index) |
|
void | notifyObserversWillRemoveProperty (Property *property, size_t index) |
|
void | notifyObserversDidRemoveProperty (Property *property, size_t index) |
|
| Observable (const Observable< PropertyOwnerObserver > &other) |
|
| Observable (Observable< PropertyOwnerObserver > &&other) |
|
Observable< PropertyOwnerObserver > & | operator= (const Observable< PropertyOwnerObserver > &other) |
|
Observable< PropertyOwnerObserver > & | operator= (Observable< PropertyOwnerObserver > &&other) |
|
void | addObserver (PropertyOwnerObserver *observer) |
|
void | removeObserver (PropertyOwnerObserver *observer) |
|
virtual void | startBlockingNotifications () override final |
|
virtual void | stopBlockingNotifications () override final |
|
| MetaDataOwner (const MetaDataOwner &rhs)=default |
|
MetaDataOwner & | operator= (const MetaDataOwner &rhs)=default |
|
void | copyMetaDataFrom (const MetaDataOwner &src) |
|
void | copyMetaDataTo (MetaDataOwner &dst) |
|
template<typename T > |
T * | createMetaData (const std::string &key) |
|
template<typename T , typename U > |
void | setMetaData (const std::string &key, U value) |
|
template<typename T > |
bool | unsetMetaData (const std::string &key) |
| unset, i.e. remove the metadata entry matching the given key and type More...
|
|
template<typename T , typename U > |
U | getMetaData (const std::string &key, U val) const |
|
template<typename T > |
T * | getMetaData (const std::string &key) |
|
template<typename T > |
const T * | getMetaData (const std::string &key) const |
|
MetaDataMap * | getMetaDataMap () |
|
const MetaDataMap * | getMetaDataMap () const |
|
template<typename T > |
bool | hasMetaData (const std::string &key) const |
|
| Observable (const Observable< ProcessorObserver > &other) |
|
| Observable (Observable< ProcessorObserver > &&other) |
|
Observable< ProcessorObserver > & | operator= (const Observable< ProcessorObserver > &other) |
|
Observable< ProcessorObserver > & | operator= (Observable< ProcessorObserver > &&other) |
|
void | addObserver (ProcessorObserver *observer) |
|
void | removeObserver (ProcessorObserver *observer) |
|
virtual void | startBlockingNotifications () override final |
|
virtual void | stopBlockingNotifications () override final |
|
|
virtual void | preProcess (TextureUnitContainer &cont) override |
| this function gets called right before the actual processing but after the shader has been activated. Overwrite this function in the derived class to perform things like custom shader setup
|
|
void | markInvalid () |
|
virtual void | postProcess () |
| this function gets called at the end of the process function Overwrite this function in the derived class to perform post-processing
|
|
virtual void | afterInportChanged () |
| this function gets called whenever the inport changes Overwrite this function in the derived class to be notified of inport onChange events
|
|
void | addPortToGroup (Port *port, const std::string &portGroup) |
|
void | removePortFromGroups (Port *port) |
|
| PropertyOwner (const PropertyOwner &rhs) |
|
PropertyOwner & | operator= (const PropertyOwner &that)=delete |
|
void | forEachObserver (C callback) |
|
void | addObservationHelper (Observer *observer) |
|
void | removeObservationHelper (Observer *observer) |
|
void | notifyObserversAboutPropertyChange (Property *p) |
|
void | notifyObserversInvalidationBegin (Processor *p) |
|
void | notifyObserversInvalidationEnd (Processor *p) |
|
void | notifyObserversIdentifierChanged (Processor *p, const std::string &oldIdentifier) |
|
void | notifyObserversDisplayNameChanged (Processor *p, const std::string &oldDisplayName) |
|
void | notifyObserversProcessorPortAdded (Processor *p, Port *port) |
|
void | notifyObserversProcessorPortRemoved (Processor *p, Port *port) |
|
void | notifyObserversAboutToProcess (Processor *p) |
|
void | notifyObserversFinishedProcess (Processor *p) |
|
void | notifyObserversSourceChange (Processor *p) |
|
void | notifyObserversSinkChange (Processor *p) |
|
void | notifyObserversReadyChange (Processor *p) |
|
void | forEachObserver (C callback) |
|
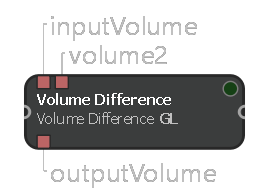
Computes the difference between two volumes by subtracting the second volume from the first one.
Inports
- inputVolume Input volume 1
- volume2 Input volume 2
Outports
- outputVolume Difference volume corresponding to
(volume 1 - volume 2 + 1.0) / 2.0